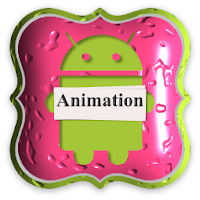
App Demo
![]() |
Tween Animation Example |
1. Creating XML-animation files
XML-animation files are usually stored in the res\anim directory. Create this directory if you don't have it in your project and then create two XML files in it: translate.xml and rotate.xml.
Type the following code into the rotate.xml file:
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:shareInterpolator="false">
<rotate
android:fromDegrees="0"
android:toDegrees="180"
android:pivotX="50%"
android:pivotY="50%"
android:duration="2500"/>
</set>
Rotate element attributes:- android:fromDegrees - starting angular position (in degrees).
- android:toDegrees - ending angular position (in degrees).
- android:pivotX - the X coordinate of the center of rotation. Expressed either in pixels relative to the object's left edge or in percentage relative to the object's left edge, or in percentage relative to the parent container's left edge.
- android:pivotY - the Y coordinate of the center of rotation. Expressed either: in pixels relative to the object's top edge, in percentage relative to the object's top edge, or in percentage relative to the parent container's top edge.
- android:duration - the amount of time for the animation to run (in milliseconds).
And now type the following code into your translate.xml file:
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:shareInterpolator="false">
<translate
android:toYDelta="-150"
android:fillAfter="true"
android:duration="1500"/>
<translate
android:toXDelta="-150"
android:fillAfter="true"
android:duration="1500"
android:startOffset="1500"/>
<translate
android:toXDelta="150"
android:fillAfter="true"
android:duration="1500"
android:startOffset="3000"/>
<translate
android:toYDelta="150"
android:fillAfter="true"
android:duration="1500"
android:startOffset="4500"/>
</set>
Translate element attributes:- android:toXDelta - ending X offset. Expressed either: in pixels relative to the normal position, in percentage relative to the element width, or in percentage relative to the parent width.
- android:toYDelta - ending Y offset. Expressed either: in pixels relative to the normal position, in percentage relative to the element height, or in percentage relative to the parent height.
- android:startOffset - the amount of milliseconds the animation delays after start() is called.
- android:duration - the amount of time for the animation to run (in milliseconds).
- android:fillAfter - when set to true, the animation transformation is applied after the animation is over.
2. Creating a shape that we will apply our animations to
The very first thing that we need to do is to create a res\drawable directory and then create shape.xml file in this directory(Right click->new Android XML file).
Now type the following code into shape.xml file:
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="#9400D3" />
</shape>
We have created a purple rectangle:)
3. Modifying activity_main.xml file
Now we need to modify our application layout file. We will add an ImageView element to place our rectangle into it and two Button elements, so that we could switch from running one animation to running the other one:)
Go to the res\layout directory and type the following code into your activity_main.xml file:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:background="#DCDCDC"
tools:context=".MainActivity" >
<ImageView
android:id="@+id/image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:minHeight="150dp"
android:minWidth="150dp"
android:layout_margin="70dp"/>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content" >
<Button
android:id="@+id/rotate"
android:layout_width="fill_parent"
android:layout_height="80dp"
android:text="@string/rotate_text"
android:layout_weight="1"/>
<Button
android:id="@+id/translate"
android:layout_width="fill_parent"
android:layout_height="80dp"
android:text="@string/translate_text"
android:layout_weight="1"/>
</TableRow>
</LinearLayout>
4. Loading Animation and Handling User Input
Our last step is to put all application elements together. Open your MainActivity.java class file and type the following code into it:
Our last step is to put all application elements together. Open your MainActivity.java class file and type the following code into it:
1| package tweenanimationexample.tuts.com;
2| import android.os.Bundle;
3| import android.app.Activity;
4| import android.content.Context;
5| import android.view.Menu;
6| import android.view.View;
7| import android.view.View.OnClickListener;
8| import android.view.animation.Animation;
9| import android.view.animation.Animation.AnimationListener;
10| import android.view.animation.AnimationUtils;
11| import android.widget.Button;
12| import android.widget.ImageView;
13| public class MainActivity extends Activity implements AnimationListener{
14| ImageView image;
15| Animation animation1;
16| Animation animation2;
17| Button rotate, translate;
18| Context context = this;
19| @Override
20| protected void onCreate(Bundle savedInstanceState) {
21| super.onCreate(savedInstanceState);
22| setContentView(R.layout.activity_main);
23| image = (ImageView) findViewById(R.id.image);
24| rotate = (Button) findViewById(R.id.rotate);
25| translate = (Button) findViewById(R.id.translate);
26| image.setImageResource(R.drawable.shape);
27| animation1 = AnimationUtils.loadAnimation(this, R.anim.rotate);
28| animation2 = AnimationUtils.loadAnimation(this, R.anim.translate);
29| userInputHandler();
30| }
31| private void userInputHandler() {
32| rotate.setOnClickListener(new OnClickListener() {
33| @Override
34| public void onClick(View v) {
35| image.startAnimation(animation1);
36| }
37| });
38| translate.setOnClickListener(new OnClickListener() {
39| @Override
40| public void onClick(View v) {
41| image.startAnimation(animation2);
42| }
43| });
44| }
45| @Override
46| public void onAnimationEnd(Animation animation) {
47| image.setVisibility(View.INVISIBLE);
48| }
49| @Override
50| public void onAnimationRepeat(Animation animation) {
51| // TODO Auto-generated method stub
52| image.setVisibility(View.VISIBLE);
53| }
54| @Override
55| public void onAnimationStart(Animation animation) {
56| // TODO Auto-generated method stub
57| image.setVisibility(View.VISIBLE);
58| }
59| }
Code explanation:
- 27-28 - here we are creating an Animation object by calling loadAnimation() method (passing the Activity context and a link to our XML-animation file to it).
- 31-44 - in the userInputHandler() method we are setting listeners on our buttons, but in the onClick() methods we are calling View.startAnimation() method to start chosen animation.
- 45-57 - AnimationListener interface implements three abstract methods that let us handle animation related events. For instance, we can make animation object invisible when the animation ends.
Source Code
1. http://developer.android.com/guide/topics/resources/animation-resource.html
2. http://developer.android.com/reference/android/view/animation/Animation.html
3. http://developer.android.com/guide/topics/resources/animation-resource.html
4. http://developer.android.com/reference/android/view/animation/Animation.AnimationListener.html