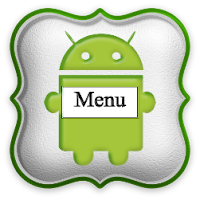
I am assuming that you already have a list of items that you will use, but if you don't you can quickly create one following the three steps of my ListView tutorial here:
1. Creating a menu and adding items to it
So the first thing that we need to do here is to create a menu and add items to it:) It is possible to create a menu through a code, but in my opinion it is easier to do it through XML file that is to be located in the res\menu directory.
Go to res\menu directory and create a new XML file (right click on menu folder New->Android XML file), add the following code to it:
<?xml
version=
"1.0"
encoding=
"utf-8"?
>
<menu
xmlns:android=
"http://schemas.android.com/apk/res/android"
>
<item
android:id=
"@+id/delete_item"
android:title=
"@string/delete_string"
/>
</menu>
As you can see I have added one item to our menu and used a string resource for its title. To create a string resource you need to go to the res\values directory, open a strings.xml file and add this tine to it:
<string
name=
"delete_string"
>
Delete
</string>
2. Registering a context menu
Go to the ActivityMain.java file and add the following line to the onCreate() method:
registerForContextMenu(getListView());
registerForContextMenu() is registering our context menu to be shown in this view. When the items of your list is clicked the registerForContextMenu() calls onCreateContextMenu() method, where your context menu is being set up. So now we need to add this method:
@Override
public void
onCreateContextMenu(ContextMenu menu, View v, ContextMenuInfo menuInfo) { // TODO Auto-generated method stub
super
.onCreateContextMenu(menu, v, menuInfo); MenuInflater m = getMenuInflater(); m.inflate(R.menu.our_context_menu, menu); }
Now that we have defined what menu is going to be inflated in the menu object we can continue to the next step.
3. Handling the item selection
To handle the selection of a context menu item add the following code:
@Override
public boolean
onContextItemSelected(MenuItem item) {
switch
(item.getItemId()){
case
R.id.delete_item: AdapterContextMenuInfo info = (AdapterContextMenuInfo) item.getMenuInfo(); position = (int) info.id; list.remove(position); this.adapter.notifyDataSetChanged(); return true; }
return
super
.
onContextItemSelected(item); }
Explanation:
This method is the one that is going to be called when a context menu items is selected. A switch statement is used to find out which item was selected. We only have one item, which is meant to delete an item from the list. To do that we perform the following actions:
- get all the information about selected item using:
AdapterContextMenuInfo info = (AdapterContextMenuInfo) item.getMenuInfo();
list.remove(position);
this.adapter.notifyDataSetChanged();
Full activity code:
package
com.example.contextmenuexample;
import
java.util.ArrayList;
import
java.util.Collections;
import
java.util.List;
import
android.os.Bundle;
import
android.app.ListActivity;
import
android.view.ContextMenu;
import
android.view.MenuInflater;
import
android.view.MenuItem;
import
android.view.View;
import
android.view.ContextMenu.ContextMenuInfo;
import
android.widget.ArrayAdapter;
import
android.widget.AdapterView.AdapterContextMenuInfo;
public class
MainActivity
extends
ListActivity {
private
String[]
items
;
private
List<String>
list
;
private
ArrayAdapter<String>
adapter
;
private int
position
; @Override
protected void
onCreate(Bundle savedInstanceState) {
super
.onCreate(savedInstanceState); setContentView(R.layout.
activity_main
); fillData(); registerForContextMenu(getListView()); }
private void
fillData() {
items
= new String[] {
"Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"
};
list
= new ArrayList<String>(); Collections.addAll(
list
,
items
);
adapter
=
new
ArrayAdapter<String>(
this
, R.layout.row, R.id.r_text, list); setListAdapter(adapter); } @Override public void onCreateContextMenu(ContextMenu menu, View v, ContextMenuInfo menuInfo) { // TODO Auto-generated method stub super.onCreateContextMenu(menu, v, menuInfo); MenuInflater m = getMenuInflater(); m.inflate(R.menu.our_context_menu, menu); } @Override public boolean onContextItemSelected(MenuItem item) { switch(item.getItemId()){ case R.id.delete_item: AdapterContextMenuInfo info = (AdapterContextMenuInfo) item.getMenuInfo(); position = (int) info.id; list.remove(position); this.adapter.notifyDataSetChanged(); return true; } return super.onContextItemSelected(item); } }
DEMO
![]() |
Android Context Menu Example Source code |